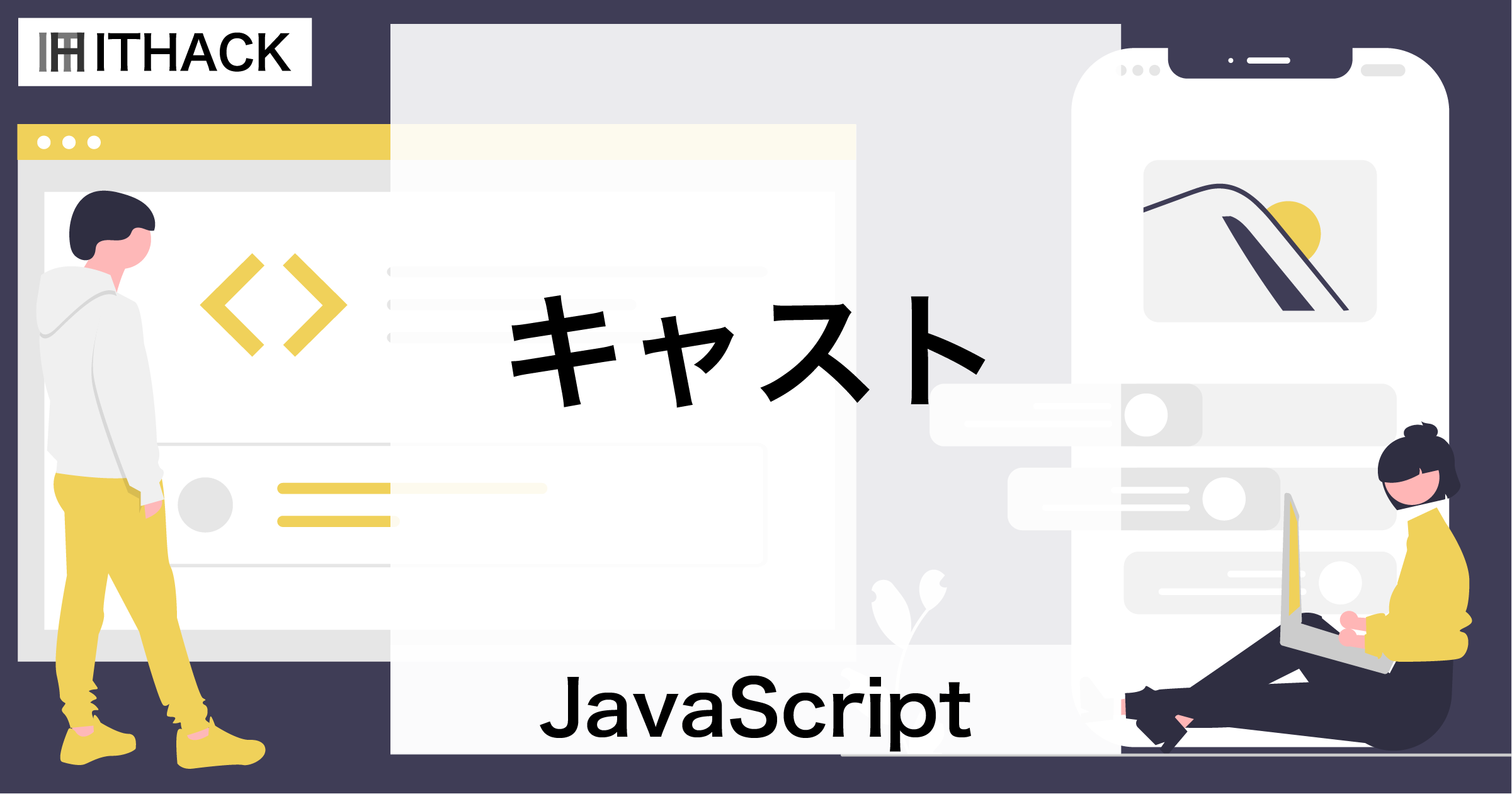
【JavaScript】キャスト(型変換) - 文字列型や数値型への変換
JavaScriptは型を意識せずにプログラミングすることができますが、意図的に型を扱えるようになると、精度の高いプログラムを作成できるようになります。
ここでは、キャスト(型変換)について解説します。
検証環境
キャスト
キャストは型変換とも呼ばれ、名前の通り“型を変換すること”です。
例えば、文字列型の1234
を数値型に変換するなどです。
数値型(number
)
数値型へのキャストはNumberを使用します。
基本構文
Number(変数または値)
丸括弧(()
)内の変数または値を数値型に変換します。
Number
は数値のラッパーオブジェクトです。
ラッパーオブジェクトが未学習の方はここでは基本構文のみ覚えていただければ問題ありません。
サンプル
文字列型 → 数値型
文字列を数値として扱える場合、数値型に変換します。
let moji = "1234";
___ih_hl_start
let num = Number(moji);
___ih_hl_end
console.log("moji (type) : " + typeof(moji));
console.log("moji (value) : " + moji);
console.log("num (type) : " + typeof(num));
console.log("num (value) : " + num);
moji (type) : string
moji (value) : 1234
num (type) : number
num (value) : 1234
文字列に数値に関する文字以外を含む場合は、NaN(Not-A-Number)という非数を表す値に変換されます。
let moji = "1234X";
___ih_hl_start
let num = Number(moji);
___ih_hl_end
console.log("moji (type) : " + typeof(moji));
console.log("moji (value) : " + moji);
console.log("num (type) : " + typeof(num));
console.log("num (value) : " + num);
moji (type) : string
moji (value) : 1234X
num (type) : number
num (value) : NaN
論理型 → 数値型
論理型の数値型へのキャストはtrue
は1
、false
は0
になります。
let bool1 = true;
___ih_hl_start
let num1 = Number(bool1);
___ih_hl_end
console.log("bool1 (type) : " + typeof(bool1));
console.log("bool1 (value) : " + bool1);
console.log("num1 (type) : " + typeof(num1));
console.log("num1 (value) : " + num1);
let bool2 = false;
___ih_hl_start
let num2 = Number(bool2);
___ih_hl_end
console.log("bool2 (type) : " + typeof(bool2));
console.log("bool2 (value) : " + bool2);
console.log("num2 (type) : " + typeof(num2));
console.log("num2 (value) : " + num2);
bool1 (type) : boolean
bool1 (value) : true
num1 (type) : number
num1 (value) : 1
bool2 (type) : boolean
bool2 (value) : false
num2 (type) : number
num2 (value) : 0
Null → 数値型
Nullの数値型へのキャストは0
になります。
let nl = null;
___ih_hl_start
let num = Number(nl);
___ih_hl_end
console.log("nl (type) : " + typeof(nl));
console.log("nl (value) : " + nl);
console.log("num (type) : " + typeof(num));
console.log("num (value) : " + num);
nl (type) : object
nl (value) : null
num (type) : number
num (value) : 0
Undefined → 数値型
Undefinedの数値型へのキャストはNaN
になります。
let ud = undefined;
___ih_hl_start
let num = Number(ud);
___ih_hl_end
console.log("ud (type) : " + typeof(ud));
console.log("ud (value) : " + ud);
console.log("num (type) : " + typeof(num));
console.log("num (value) : " + num);
ud (type) : undefined
ud (value) : undefined
num (type) : number
num (value) : NaN
文字列型(string
)
文字列型へのキャストはStringを使用します。
基本構文
String(変数または値)
丸括弧(()
)内の変数または値を文字列型に変換します。
String
は文字列のラッパーオブジェクトです。
ラッパーオブジェクトが未学習の方はここでは基本構文のみ覚えていただければ問題ありません。
サンプル
数値型 → 文字列型
数値型の文字列型へのキャストは値をそのまま文字列に変換します。
let num = 1234;
___ih_hl_start
let moji = String(num);
___ih_hl_end
console.log("num (type) : " + typeof(num));
console.log("num (value) : " + num);
console.log("moji (type) : " + typeof(moji));
console.log("moji (value) : " + moji);
num (type) : number
num (value) : 1234
moji (type) : string
moji (value) : 1234
論理型 → 文字列型
論理型の文字列型へのキャストはtrue
/false
をそのまま文字列に変換します。
let bool1 = true;
___ih_hl_start
let moji1 = String(bool1);
___ih_hl_end
console.log("bool1 (type) : " + typeof(bool1));
console.log("bool1 (value) : " + bool1);
console.log("moji1 (type) : " + typeof(moji1));
console.log("moji1 (value) : " + moji1);
let bool2 = false;
___ih_hl_start
let moji2 = String(bool2);
___ih_hl_end
console.log("bool2 (type) : " + typeof(bool2));
console.log("bool2 (value) : " + bool2);
console.log("moji2 (type) : " + typeof(moji2));
console.log("moji2 (value) : " + moji2);
bool1 (type) : boolean
bool1 (value) : true
moji1 (type) : string
moji1 (value) : true
bool2 (type) : boolean
bool2 (value) : false
moji2 (type) : string
moji2 (value) : false
Null → 文字列型
Nullの文字列型へのキャストは値をそのまま文字列に変換します。
let nl = null;
___ih_hl_start
let moji = String(nl);
___ih_hl_end
console.log("nl (type) : " + typeof(nl));
console.log("nl (value) : " + nl);
console.log("moji (type) : " + typeof(moji));
console.log("moji (value) : " + moji);
nl (type) : object
nl (value) : null
moji (type) : string
moji (value) : null
Undefined → 文字列型
Undefinedの文字列型へのキャストは値をそのまま文字列に変換します。
let ud = undefined;
___ih_hl_start
let moji = String(ud);
___ih_hl_end
console.log("ud (type) : " + typeof(ud));
console.log("ud (value) : " + ud);
console.log("moji (type) : " + typeof(moji));
console.log("moji (value) : " + moji);
ud (type) : undefined
ud (value) : undefined
moji (type) : string
moji (value) : undefined
論理型(boolean
)
文字列型へのキャストはBooleanを使用します。
基本構文
Boolean(変数または値)
丸括弧(()
)内の変数または値を論理型に変換します。
Boolean
は論理値のラッパーオブジェクトです。
ラッパーオブジェクトが未学習の方はここでは基本構文のみ覚えていただければ問題ありません。
サンプル
数値型 → 論理型
数値型の論理型へのキャストは0
以外はtrue
、0
はfalse
に変換します。
let num1 = 1;
___ih_hl_start
let bool1 = Boolean(num1);
___ih_hl_end
console.log("num1 (type) : " + typeof(num1));
console.log("num1 (value) : " + num1);
console.log("bool1 (type) : " + typeof(bool1));
console.log("bool1 (value) : " + bool1);
let num2 = 0;
___ih_hl_start
let bool2 = Boolean(num2);
___ih_hl_end
console.log("num2 (type) : " + typeof(num2));
console.log("num2 (value) : " + num2);
console.log("bool2 (type) : " + typeof(bool2));
console.log("bool2 (value) : " + bool2);
num1 (type) : number
num1 (value) : 1
bool1 (type) : boolean
bool1 (value) : true
num2 (type) : number
num2 (value) : 0
bool2 (type) : boolean
bool2 (value) : false
文字列 → 論理型
文字列型の論理型へのキャストは空文字以外はtrue
、空文字はfalse
に変換します。
let moji1 = "ABC";
___ih_hl_start
let bool1 = Boolean(moji1);
___ih_hl_end
console.log("moji1 (type) : " + typeof(moji1));
console.log("moji1 (value) : " + moji1);
console.log("bool1 (type) : " + typeof(bool1));
console.log("bool1 (value) : " + bool1);
let moji2 = "";
___ih_hl_start
let bool2 = Boolean(moji2);
___ih_hl_end
console.log("moji2 (type) : " + typeof(moji2));
console.log("moji2 (value) : " + moji2);
console.log("bool2 (type) : " + typeof(bool2));
console.log("bool2 (value) : " + bool2);
moji1 (type) : string
moji1 (value) : ABC
bool1 (type) : boolean
bool1 (value) : true
moji2 (type) : string
moji2 (value) :
bool2 (type) : boolean
bool2 (value) : false
Null → 論理型
Nullの論理型へのキャストはfalse
に変換します。
let nl = null;
___ih_hl_start
let bool = Boolean(nl);
___ih_hl_end
console.log("nl (type) : " + typeof(nl));
console.log("nl (value) : " + nl);
console.log("bool (type) : " + typeof(bool));
console.log("bool (value) : " + bool);
nl (type) : object
nl (value) : null
bool (type) : boolean
bool (value) : false
Undefined → 論理型
Undefinedの論理型へのキャストはfalse
に変換します。
let ud = undefined;
___ih_hl_start
let bool = Boolean(ud);
___ih_hl_end
console.log("ud (type) : " + typeof(ud));
console.log("ud (value) : " + ud);
console.log("bool (type) : " + typeof(bool));
console.log("bool (value) : " + bool);
ud (type) : undefined
ud (value) : undefined
bool (type) : boolean
bool (value) : false