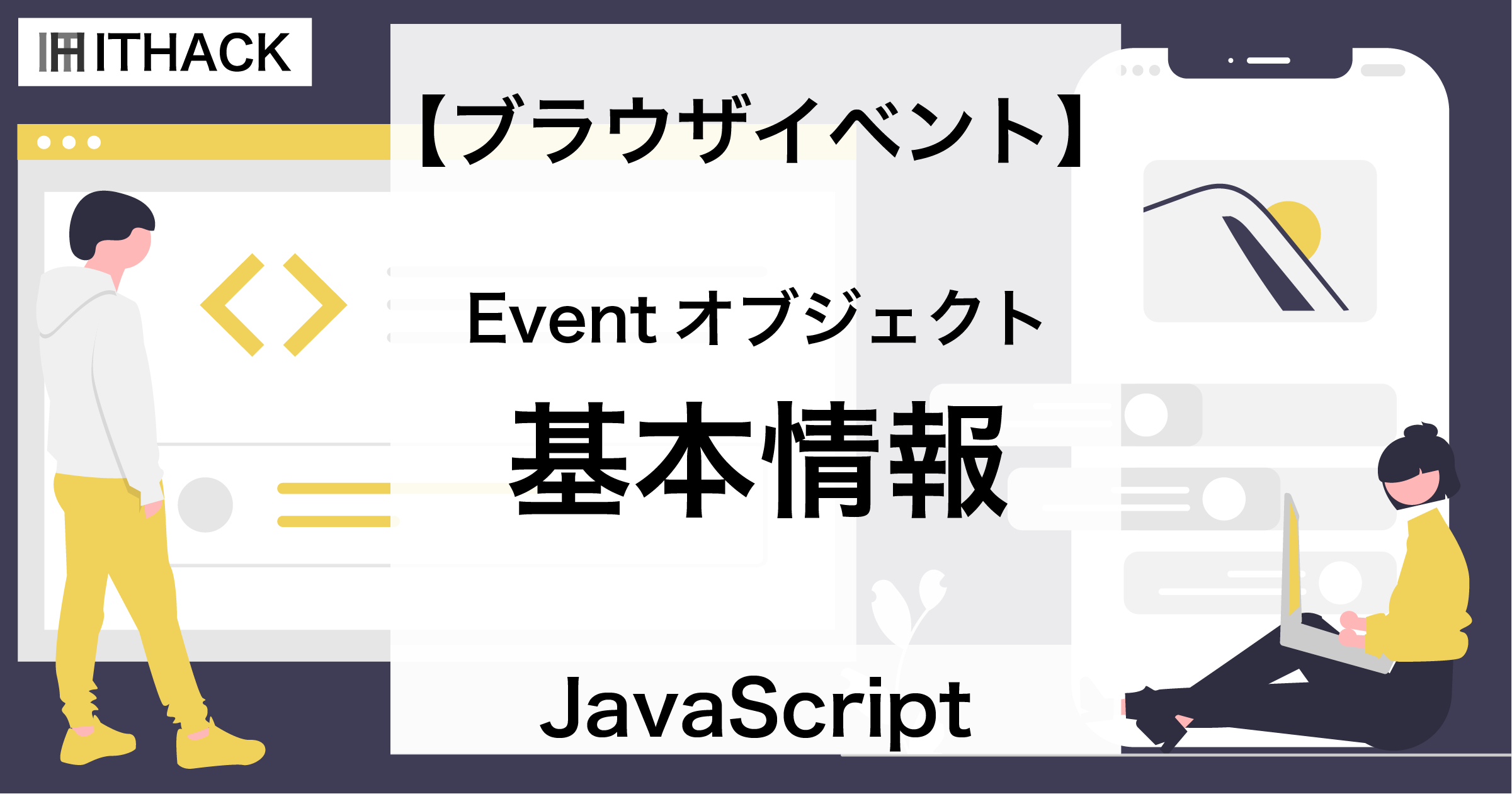
【JavaScript】Eventオブジェクト - 基本情報 / イベント情報をコールバック関数で受け取る
JavaScript/ブラウザイベントの『Eventオブジェクトの基本情報』について解説します。
検証環境
Eventオブジェクト
Eventオブジェクトは“イベントの情報を表すオブジェクト”です。
イベントハンドラーのコールバック関数に第1引数(仮引数)を定義した場合、自動で実行時に与えられます。
次のサンプルコードで確認します。
<button id="btn">ボタン</button>
<script type="text/javascript">
___ih_hl_start
function output( event ) {
___ih_hl_end
console.log(event);
}
let btn = document.getElementById('btn');
btn.addEventListener('click', output);
</script>
PointerEvent {isTrusted: true, pointerId: 1, width: 1, height: 1, pressure: 0, …}
output
関数は第1引数に仮引数event
を定義しています。
このようにすることで、イベント発生に伴う実行時に仮引数event
に自動でEventオブジェクトが与えられます。
※ このサンプルコードはブラウザ表示し、ボタンをクリックすると、コンソールにEventオブジェクトを出力します。
Eventオブジェクトの基本的なプロパティについて以下で解説します。
プロパティ
Eventオブジェクトはイベントに関する様々なプロパティを所有します。
target
target
プロパティはイベント発生源の要素です。
<button id="btn">ボタン</button>
<script type="text/javascript">
function output( event ) {
___ih_hl_start
console.log(event.target);
___ih_hl_end
}
let btn = document.getElementById('btn');
btn.addEventListener('click', output);
</script>
このサンプルコードをブラウザで実行し、ボタンをクリックすると、次のようにbutton[id="btn"]
要素をコンソールに出力します。
<button id="btn">ボタン</button>
type
type
プロパティはイベント種別です。
<button id="btn">ボタン</button>
<script type="text/javascript">
function output( event ) {
___ih_hl_start
console.log(event.type);
___ih_hl_end
}
let btn = document.getElementById('btn');
btn.addEventListener('click', output);
</script>
このサンプルコードをブラウザで実行し、ボタンをクリックすると、次のようなコンソール結果になります。
click
isTrusted
isTrusted
プロパティはユーザー操作によるイベント発生の可否です。
論理値になっており、true
はユーザー操作によるイベントとみなされたことを表し、false
の場合はそれ以外を表します。
<button id="btn">ボタン</button>
<script type="text/javascript">
function output( event ) {
console.log(event.isTrusted);
}
let btn = document.getElementById('btn');
btn.addEventListener('click', output);
</script>
このサンプルコードをブラウザで実行し、ボタンをクリックすると、次のようなコンソール結果になります。
true
timeStamp
timeStamp
プロパティはイベント生成時刻(ミリ秒)です。
<button id="btn">ボタン</button>
<script type="text/javascript">
function output(event) {
___ih_hl_start
console.log(event.timeStamp);
___ih_hl_end
}
let btn = document.getElementById('btn');
btn.addEventListener('click', output);
</script>
このサンプルコードをブラウザで実行し、ボタンをクリックすると、次のようなコンソール結果になります。
1073.6000001430511
※ この数値はイベント生成時刻を表すため、ボタンをクリックした時刻によって異なります。
clientX / clientY
clientX
プロパティとclientY
プロパティはウィンドウ上のマウスカーソルの位置です。
<button id="btn">ボタン</button>
<script type="text/javascript">
function output(event) {
___ih_hl_start
console.log(event.clientX, event.clientY);
___ih_hl_end
}
let btn = document.getElementById('btn');
btn.addEventListener('click', output);
</script>
このサンプルコードをブラウザで実行し、ボタンをクリックすると、次のようなコンソール結果になります。
41 16
※ この数値はウィンドウ上のマウスカーソルの位置を表すため、実行環境により異なります。